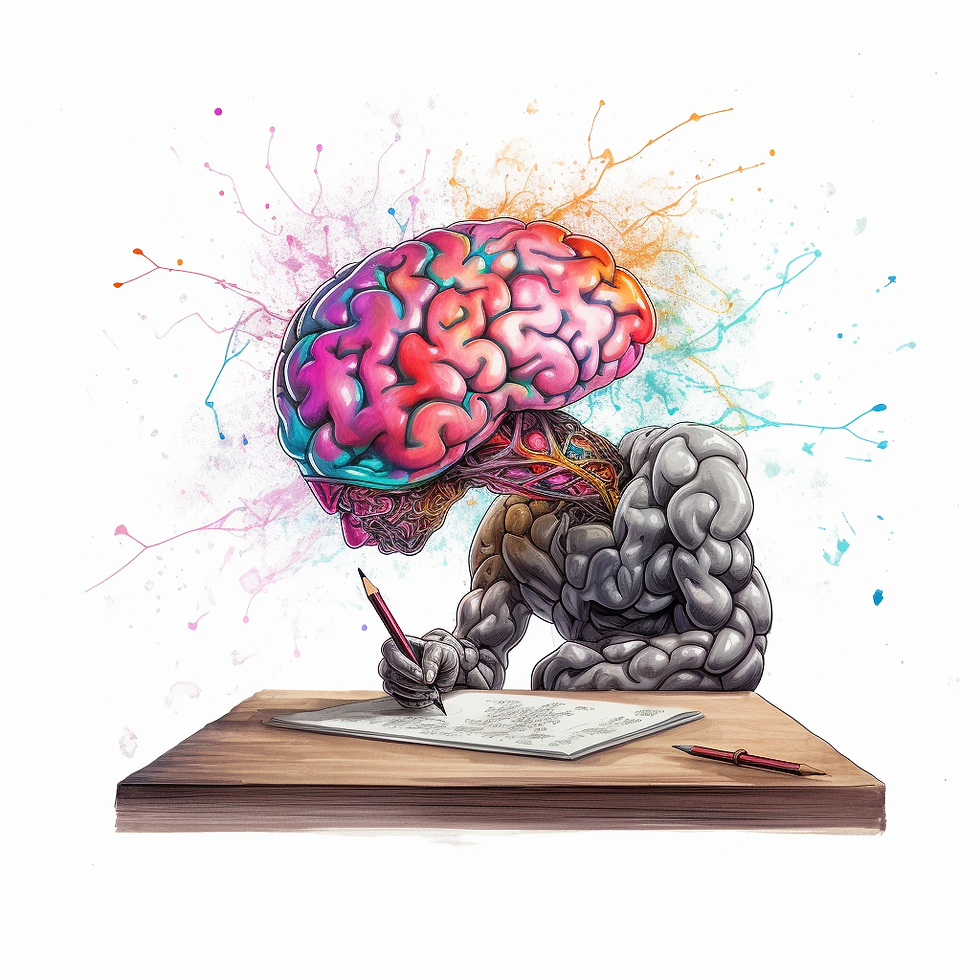
In the dynamic realm of blockchain technology, Decentralized Autonomous Organizations, better known as DAOs, have paved the way for a new form of democratic governance, enabling collective decision-making in a decentralized manner. At the heart of these DAOs lie smart contracts, particularly those written in Solidity, the primary language for Ethereum based contracts.
Today, we'll delve into a rudimentary DAO modeled via a Solidity smart contract. As we go along, we'll deconstruct its building blocks and offer a glimpse of how such a contract can automate organizational processes in a decentralized context.
Before we get started, here's the contract that we'll be dissecting:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleDAO {
struct Proposal {
string description;
uint voteCount;
}
address public manager;
mapping(address => bool) public members;
Proposal[] public proposals;
constructor() {
manager = msg.sender;
}
function join() public {
// Everyone can join this DAO by calling this function
members[msg.sender] = true;
}
function propose(string memory description) public {
// Only members can propose
require(members[msg.sender], "Only members can propose");
Proposal memory newProposal = Proposal({
description: description,
voteCount: 0
});
proposals.push(newProposal);
}
function vote(uint index) public {
// Only members can vote
require(members[msg.sender], "Only members can vote");
// Increase the vote count of a proposal
Proposal storage proposal = proposals[index];
proposal.voteCount += 1;
}
}
This basic DAO contract, written in Solidity, encapsulates the core elements of a DAO. It offers a platform for anyone to become a member, suggest ideas or proposals, and participate in the decision-making process by voting on these proposals. Let's dive in and understand each component.
The Structure: The contract starts with the definition of a Proposal struct that has two properties: description (to store the details of the proposal) and voteCount (to keep track of the votes each proposal receives). Next, we define the state variables: manager (the contract creator), members (a mapping to store the membership status of an address), and proposals (an array to hold all proposals).
Initialization: The constructor function is automatically executed upon contract deployment. Here, we set the manager to the address deploying the contract, meaning the one who instantiates the DAO.
Joining the DAO: The join() function facilitates the inclusion of new members. Anyone can join the DAO simply by calling this function, which then sets their membership status to true in the members mapping.
Proposing an Idea: The propose() function allows any member of the DAO to propose new ideas. Each proposal is encapsulated as a Proposal struct and is appended to the proposals array. The require statement preceding the function ensures that only members can create proposals.
Voting on Proposals: The vote() function empowers members to vote on a proposal by specifying its index. With each vote, the chosen proposal's voteCount increases by one. Again, the require statement confirms that only members can vote.
At this point, it's crucial to note that this contract, while a valid illustration, is significantly simplified. It doesn't cater to several real-world conditions essential for DAO operations such as quorum requirements, voting timeframes, proposal execution, and safety mechanisms against malicious activities.
Creating a functional DAO involves a robust understanding of blockchain security practices and a complex blend of social, economic, and technical design decisions. Contracts intended for real-world use must be exhaustively audited to ensure their security and effectiveness.
This basic DAO contract, however, serves as a starting point for grasping the potential of DAOs and the transformative power of Ethereum. DAOs herald an exciting future, enabling novel ways for communities to self-govern and collaborate. Solidity, being the crux of such decentralized systems, is an invaluable tool for anyone eager to contribute to this evolving ecosystem.
Disclaimer: The contract provided is a simplified version intended only for educational purposes and should not be deployed for real-world applications as it lacks several critical features and security measures. Always consult with professionals when dealing with smart contracts and actual assets.
Comments